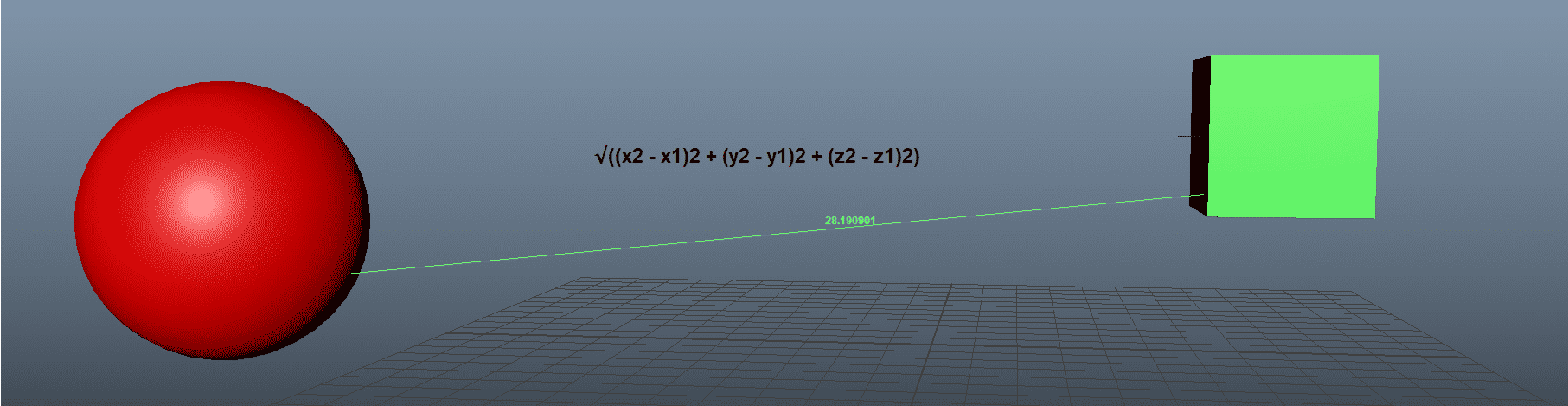
Measure distance with OpenMaya
Recently I received a request from a layout artist, he asked me about a script to get the distance from the camera to any selected object in the Maya viewport. His specific case was to set the depth of field of the camera based on the distance value.
His second request was for the script to be able to be mapped into a hotkey for quick access.
While there are several ways to approach this request, I decided to build a small tool to solve this issue using OpenMaya instead of cmds. If you are here just looking for the tool, you can find it for free here: CalculateDistance
If you are more curious and want to have a sneak peak of the code, here is a python snippet showing how to get the distance between the current active camera and any selected object’s pivot point.
This snippet only prints the distance from the camera to the object’s pivot (worldspace xform), while the full version of the tool has this extra features:
- Allows to get the closest point from the camera to the surface of a geometry. (Vertex based instead of xform)
- Create a locator in the closest point on the surface of the object.
- Measure distance between 2 selected objects.
- Shows a prompt box to copy the distance without opening the script editor.
To test this snippet, select any object (geometry, locator, group, etc), and run it.
# Imports import maya.OpenMayaUI as omui import maya.OpenMaya as om import maya.cmds as cmds from math import pow,sqrt # Get selection from vieport getSelection=cmds.ls(selection=True) # Get current active camera view = omui.M3dView.active3dView() cam = om.MDagPath() view.getCamera(cam) # Get the parent of the camera shape getCamera = cmds.listRelatives(cam.fullPathName(), p=True) # Wodlspace xform of the camera camXForm = cmds.xform(getCamera[0], q=True, t=True, ws=True) # Worldspace xform of the selected object objXform = cmds.xform(getSelection[0], q=True, t=True, ws=True) # Distance formula : √((x2 - x1)2 + (y2 - y1)2 + (z2 - z1)2) distance = sqrt(pow(camXForm[0]-objXform[0],2)+pow(camXForm[1]-objXform[1],2)+pow(camXForm[2]-objXform[2],2)) # Just print the distance print distance
If you find this useful, please leave a comment with some feedback or requests!